Let's Create a REST API With Spring Boot
In this post, we will learn how to create REST API with Spring Boot, JPA, Hibernate, and MySQL.
- Create the Spring Boot Project.
- Define Database configurations.
- Create an Entity Class.
- Create JPA Data Repository layer.
- Create Rest Controllers and map API requests.
- Create Unit Testing for API requests and run the unit testing.
- Build and run the Project.
Requirement
We need to create a simple REST API to store user data to MySQL database with that API so we can create, update, delete, and get users information.
Create the Spring Boot Project
First, go to Spring Initializr and create a project with below settings
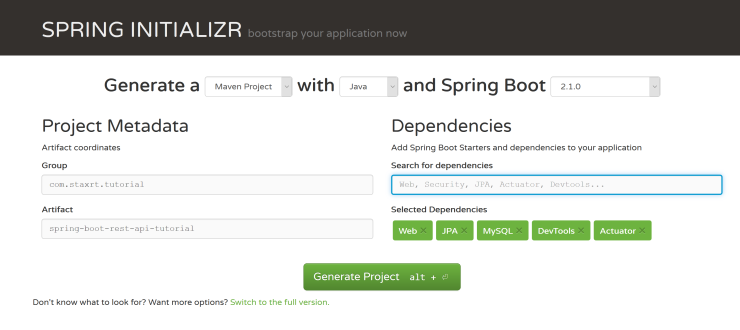
Web — Full-stack web development with Tomcat and Spring MVC
DevTools — Spring Boot Development Tools
JPA — Java Persistence API including spring-data-JPA, spring-orm, and Hibernate
MySQL — MySQL JDBC driver
Actuator — Production-ready features to help you monitor and manage your application
Generate, download, and import to development IDE.
Define Database Configurations
Next Create the database name called user_database in MySQL database server and define connection properties in spring-boot-rest-api-tutorial/src/main/resources/application.properties
## Database Properties
spring.datasource.url = jdbc:mysql://localhost:3306/users_database?useSSL=false
spring.datasource.username = root
spring.datasource.password = root
## Hibernate Properties
# The SQL dialect makes Hibernate generate better SQL for the chosen database
spring.jpa.properties.hibernate.dialect = org.hibernate.dialect.MySQL5InnoDBDialect
# Hibernate ddl auto (create, create-drop, validate, update)
spring.jpa.hibernate.ddl-auto = update
Create Entity Class
@Entity
@Table(name = "users")
@EntityListeners(AuditingEntityListener.class)
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private long id;
@Column(name = "first_name", nullable = false)
private String firstName;
@Column(name = "last_name", nullable = false)
private String lastName;
@Column(name = "email_address", nullable = false)
private String email;
@Column(name = "created_at", nullable = false)
@CreatedDate
private Date createdAt;
@Column(name = "created_by", nullable = false)
@CreatedBy
private String createdBy;
@Column(name = "updated_at", nullable = false)
@LastModifiedDate
private Date updatedAt;
@Column(name = "updated_by", nullable = false)
@LastModifiedBy
private String updatedBy;
/**
* Gets id.
*
* @return the id
*/
public long getId() {
return id;
}
/**
* Sets id.
*
* @param id the id
*/
public void setId(long id) {
this.id = id;
}
/**
* Gets first name.
*
* @return the first name
*/
public String getFirstName() {
return firstName;
}
/**
* Sets first name.
*
* @param firstName the first name
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
* Gets last name.
*
* @return the last name
*/
public String getLastName() {
return lastName;
}
/**
* Sets last name.
*
* @param lastName the last name
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
* Gets email.
*
* @return the email
*/
public String getEmail() {
return email;
}
/**
* Sets email.
*
* @param email the email
*/
public void setEmail(String email) {
this.email = email;
}
/**
* Gets created at.
*
* @return the created at
*/
public Date getCreatedAt() {
return createdAt;
}
/**
* Sets created at.
*
* @param createdAt the created at
*/
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
/**
* Gets created by.
*
* @return the created by
*/
public String getCreatedBy() {
return createdBy;
}
/**
* Sets created by.
*
* @param createdBy the created by
*/
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
/**
* Gets updated at.
*
* @return the updated at
*/
public Date getUpdatedAt() {
return updatedAt;
}
/**
* Sets updated at.
*
* @param updatedAt the updated at
*/
public void setUpdatedAt(Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
* Gets updated by.
*
* @return the updated by
*/
public String getUpdatedBy() {
return updatedBy;
}
/**
* Sets updated by.
*
* @param updatedBy the updated by
*/
public void setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
}
}
Create JPA Data Repository Layer
@Repository
public interface UserRepository extends JpaRepository<User, Long> {}
Create Rest Controllers and Map API Requests
@RestController
@RequestMapping("/api/v1")
public class UserController {
@Autowired
private UserRepository userRepository;
/**
* Get all users list.
*
* @return the list
*/
@GetMapping("/users")
public List<User> getAllUsers() {
return userRepository.findAll();
}
/**
* Gets users by id.
*
* @param userId the user id
* @return the users by id
* @throws ResourceNotFoundException the resource not found exception
*/
@GetMapping("/users/{id}")
public ResponseEntity<User> getUsersById(@PathVariable(value = "id") Long userId)
throws ResourceNotFoundException {
User user =
userRepository
.findById(userId)
.orElseThrow(() -> new ResourceNotFoundException("User not found on :: " + userId));
return ResponseEntity.ok().body(user);
}
/**
* Create user user.
*
* @param user the user
* @return the user
*/
@PostMapping("/users")
public User createUser(@Valid @RequestBody User user) {
return userRepository.save(user);
}
/**
* Update user response entity.
*
* @param userId the user id
* @param userDetails the user details
* @return the response entity
* @throws ResourceNotFoundException the resource not found exception
*/
@PutMapping("/users/{id}")
public ResponseEntity<User> updateUser(
@PathVariable(value = "id") Long userId, @Valid @RequestBody User userDetails)
throws ResourceNotFoundException {
User user =
userRepository
.findById(userId)
.orElseThrow(() -> new ResourceNotFoundException("User not found on :: " + userId));
user.setEmail(userDetails.getEmail());
user.setLastName(userDetails.getLastName());
user.setFirstName(userDetails.getFirstName());
user.setUpdatedAt(new Date());
final User updatedUser = userRepository.save(user);
return ResponseEntity.ok(updatedUser);
}
/**
* Delete user map.
*
* @param userId the user id
* @return the map
* @throws Exception the exception
*/
@DeleteMapping("/user/{id}")
public Map<String, Boolean> deleteUser(@PathVariable(value = "id") Long userId) throws Exception {
User user =
userRepository
.findById(userId)
.orElseThrow(() -> new ResourceNotFoundException("User not found on :: " + userId));
userRepository.delete(user);
Map<String, Boolean> response = new HashMap<>();
response.put("deleted", Boolean.TRUE);
return response;
}
}
Create Unit Testing for API Requests and Run the Unit Testing
@RunWith(SpringRunner.class)
@SpringBootTest(classes = Application.class, webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class ApplicationTests {
@Autowired
private TestRestTemplate restTemplate;
@LocalServerPort
private int port;
private String getRootUrl() {
return "http://localhost:" + port;
}
@Test
public void contextLoads() {
}
@Test
public void testGetAllUsers() {
HttpHeaders headers = new HttpHeaders();
HttpEntity<String> entity = new HttpEntity<String>(null, headers);
ResponseEntity<String> response = restTemplate.exchange(getRootUrl() + "/users",
HttpMethod.GET, entity, String.class);
Assert.assertNotNull(response.getBody());
}
@Test
public void testGetUserById() {
User user = restTemplate.getForObject(getRootUrl() + "/users/1", User.class);
System.out.println(user.getFirstName());
Assert.assertNotNull(user);
}
@Test
public void testCreateUser() {
User user = new User();
user.setEmail("admin@gmail.com");
user.setFirstName("admin");
user.setLastName("admin");
user.setCreatedBy("admin");
user.setUpdatedBy("admin");
ResponseEntity<User> postResponse = restTemplate.postForEntity(getRootUrl() + "/users", user, User.class);
Assert.assertNotNull(postResponse);
Assert.assertNotNull(postResponse.getBody());
}
@Test
public void testUpdatePost() {
int id = 1;
User user = restTemplate.getForObject(getRootUrl() + "/users/" + id, User.class);
user.setFirstName("admin1");
user.setLastName("admin2");
restTemplate.put(getRootUrl() + "/users/" + id, user);
User updatedUser = restTemplate.getForObject(getRootUrl() + "/users/" + id, User.class);
Assert.assertNotNull(updatedUser);
}
@Test
public void testDeletePost() {
int id = 2;
User user = restTemplate.getForObject(getRootUrl() + "/users/" + id, User.class);
Assert.assertNotNull(user);
restTemplate.delete(getRootUrl() + "/users/" + id);
try {
user = restTemplate.getForObject(getRootUrl() + "/users/" + id, User.class);
} catch (final HttpClientErrorException e) {
Assert.assertEquals(e.getStatusCode(), HttpStatus.NOT_FOUND);
}
}
}
Build and Run the Project
mvn package
java -jar target/spring-boot-rest-api-tutorial-1.0.0.jar
Alternatively, you can run the app without packaging it using:
mvn spring-boot:run
The app will start running at http://localhost:8080.
- Get link
- X
- Other Apps
- Get link
- X
- Other Apps
Comments
Post a Comment